Of course I found an answer to this in a collection of Stack Overflow questions, but to make things easier for anybody who might stumble into this post (and mainly for Future Me), here’s the answer.
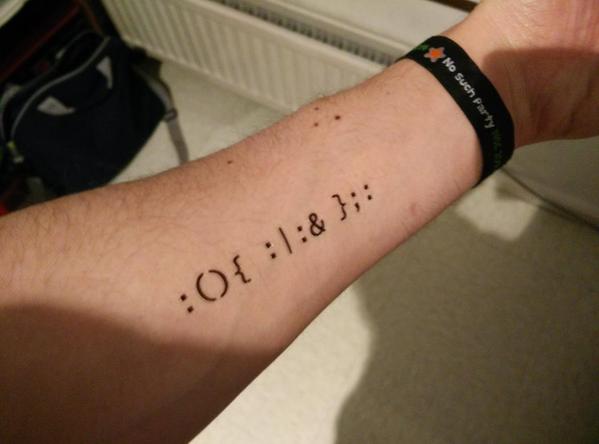
Basically I have a command that sometimes produces an error, but usually just re-running it produces the correct output. When it encounters an error, it dutifully sets its error code to something other than 0 (success). But how to I capture both the command’s output and its exit code? This way.
output=$(myCommandWhichSometimesDoesntWork) exit_code=$? # This HAS to be exeuted right after the command above |
So I made a little wrapper script that repeatedly calls the first one until it gets an answer, with a set maximum number of retries to avoid infinite loops.
#!/bin/bash retries=0 max_retries=10 # Change this appropriately # The "2> /dev/null" silences stderr redirecting it to /dev/null # The command must be first executed outside of the while loop: # bash does not have a do...while construct output=$(myCommandWhichSometimesDoesntWork 2> /dev/null) while [[ $? -gt 0 ]] do ((retries++)) if [[ retries -gt max_retries ]] then exit 1 fi output=$(myCommandWhichSometimesDoesntWork 2> /dev/null) done echo $output |